Control a DC motor from the keyboard with the TB6612FNG driver and the BeagleBone Black
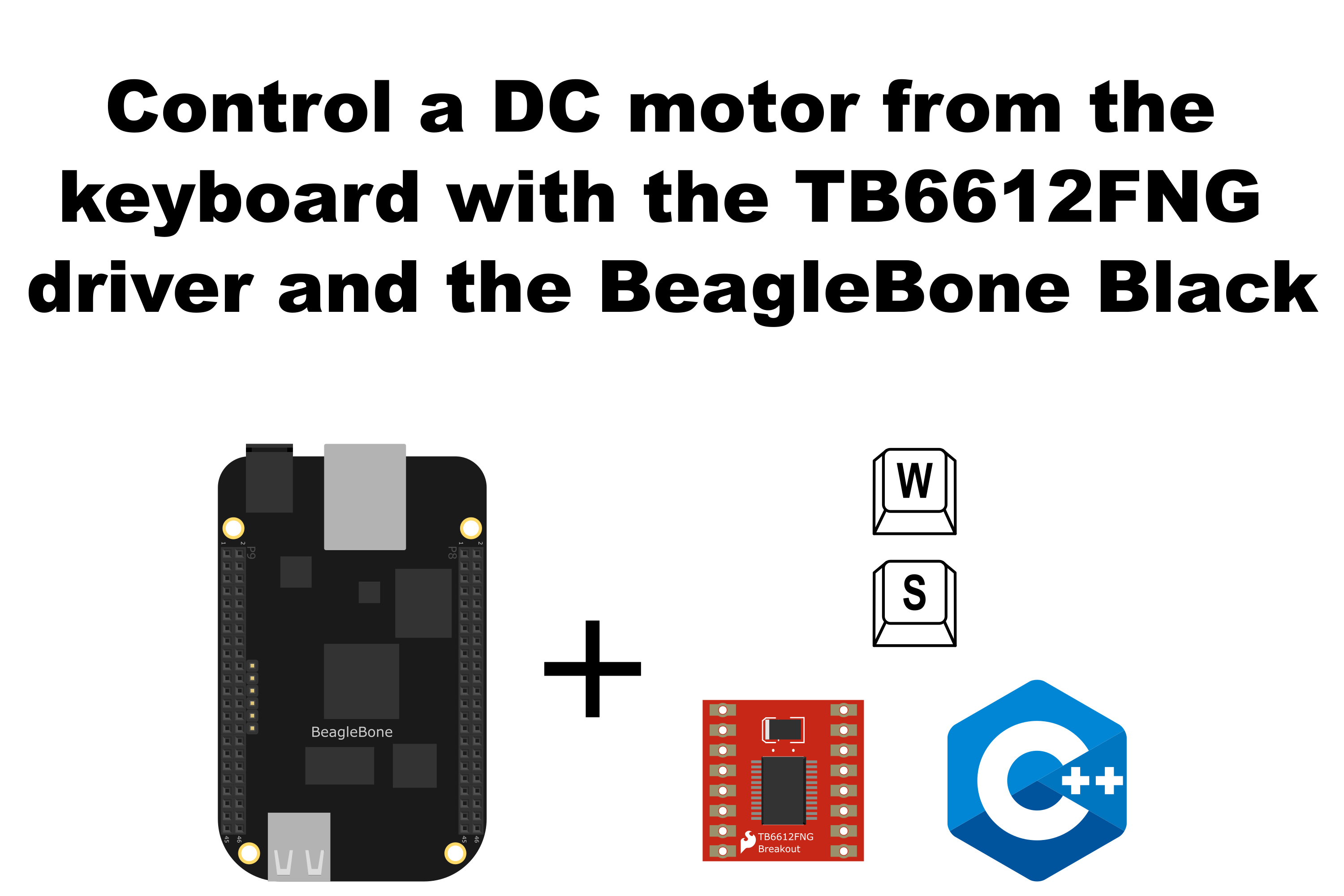
In this post, I show how to drive a DC Motor from the keyboard using the BeagleBone and the TB6612FNG driver from Toshiba. In the last entry, you can read about how to drive a motor with this driver.
It is important to remember that the logic voltage for the BeagleBone is 3.3V. If the user provides a greater voltage, the BeagleBone could be damaged.
Circuit and components
The circuit can be seen in Figure 1. It consists of a TB6612FNG driver, a low voltage DC Motor, 4 AA batteries, and the BeagleBone.
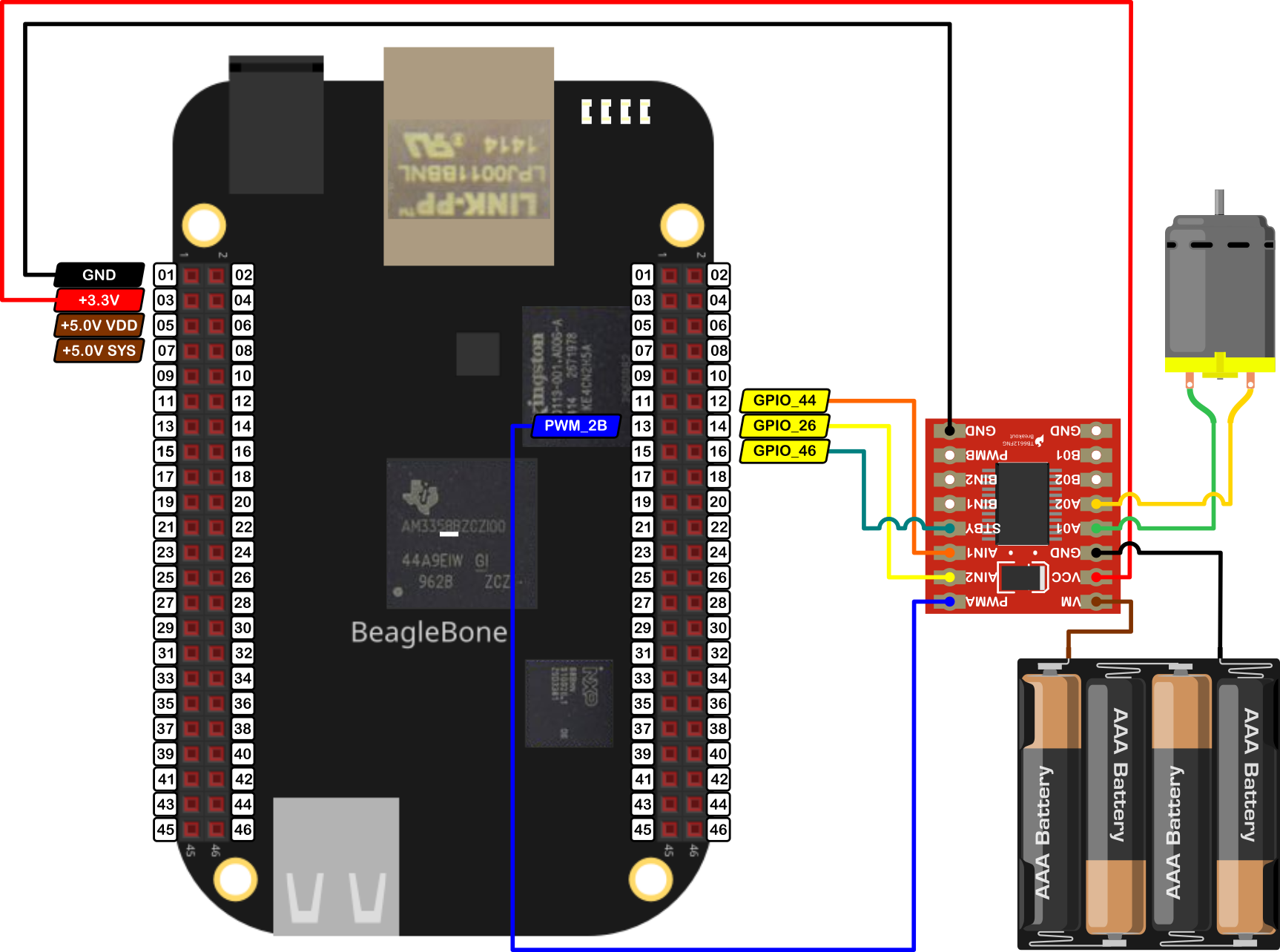
The components are:
- 1 DC Motor driver TB6612FNG
- 1 DC Motor 3.0 - 6.0V
- 4 AA Batteries
- 1 Protoboard mini
- Jumpers male-male to make the connections
The pins used for control the motor are:
- GPIO P8_12 and P8_14 to control the motor rotation direction
- PWM P8_13 to control the speed
- GPIO P8_16 to activate / deactivate de driver
Coding
First, some GPIO
and TB6612FNG
objects are declared with global scope to initialize the digital pin to activate/deactivate the driver, the control pins, and the Motor that will be driven. These GPIO
objects are used as the parameters to construct the TB6612FNG
object.
Remember that the user can include a boolean initialization parameter true/false
to invert by software the motor rotation direction instead of inverting the motor’s jumpers physically.
1
2
3
4
5
6
7
8
9
10
// Declare the pin to activate / deactivate the TB6612FNG module
GPIO standByPin(P8_16);
// Declaring the pins for motor
GPIO AIN1 (P8_12);
GPIO AIN2 (P8_14);
PWM PWMA (P8_13);
// Declare the motor object
TB6612FNG MotorA (AIN1, AIN2, PWMA, false);
The first thing to do is to activate the TB6612FNG driver. This can be done with the next line:
1
2
// Activate the module
ActivateTB6612FNG(standByPin);
Next, the user can change the motor speed using the key “W” and “S” to increase or decrease it, respectively. The class method DCMotor::Drive()
, checks if the user has input a value for the speed beyond the limits of 100 and -100` and keep it between this range, but in this case, the user code can do that too. If the user presses the key “Y”, the program finishes.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
message = "Enter an option 'y', 'w', 's': ";
cout << RainbowText(message, "Yellow");
cin >> userInput;
switch (userInput)
{
case 'w':
motorSpeed += 10;
if (motorSpeed >= 100)
motorSpeed = 100;
break;
case 's':
motorSpeed -= 10;
if (motorSpeed <= -100)
motorSpeed = -100;
break;
default:
break;
}
The instruction to move the motors is MotorA.Drive(i);
which is the core class method that receives as an argument the speed value and depending on that, it configures the desired rotation direction and speed for the motor.
1
2
// Move the motor
MotorA.Drive(motorSpeed);
Finally, the TB6612FNG has to be deactivated using the next line.
1
2
// Deactivate the module
DeactivateTB6612FNG(standByPin);
The code uses a while loop
to drive the motor increasing and decreasing the speed until the user press the key “Y”. This is shown in the next listing together with its corresponding execution output.
TB6612FNG_1.2.cpp
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
/******************************************************************************
TB6612FNG_1.2.cpp
@wgaonar
04/07/2021
https://github.com/wgaonar/BeagleCPP
- Control de motor speed with 'w' and 's' keys while the user does not press 'y'
Class: TB6612FNG
******************************************************************************/
#include <iostream>
#include "../../../Sources/TB6612FNG.h"
using namespace std;
// Declare the pin to activate / deactivate the TB6612FNG module
GPIO standByPin(P8_16);
// Declaring the pins for motor
GPIO AIN1 (P8_12);
GPIO AIN2 (P8_14);
PWM PWMA (P8_13);
// Declare the motor object
TB6612FNG MotorA (AIN1, AIN2, PWMA, false);
int main()
{
// Activate the module
ActivateTB6612FNG(standByPin);
string message = "Main program starting here...";
cout << RainbowText(message,"Blue", "White", "Bold") << endl;
message = "If you want to stop the program, enter 'y' for yes";
cout << RainbowText(message, "Yellow") << endl;
message = "Or enter 'w' for increase speed or 's' for decrease it";
cout << RainbowText(message, "Yellow") << endl;
int motorSpeed = 0;
char userInput = '\0';
while (userInput != 'y')
{
message = "Enter an option 'y', 'w', 's': ";
cout << RainbowText(message, "Yellow");
cin >> userInput;
switch (userInput)
{
case 'w':
motorSpeed += 10;
if (motorSpeed >= 100)
motorSpeed = 100;
break;
case 's':
motorSpeed -= 10;
if (motorSpeed <= -100)
motorSpeed = -100;
break;
default:
break;
}
// Move the motor
MotorA.Drive(motorSpeed);
}
// Deactivate the module
DeactivateTB6612FNG(standByPin);
message = "Main program finishes here...";
cout << RainbowText(message,"Blue", "White","Bold") << endl;
return 0;
}
Execution of the program:
Se you in the next post.