Control the Brightness of a LED with a Potentiometer in the BeagleBone Black
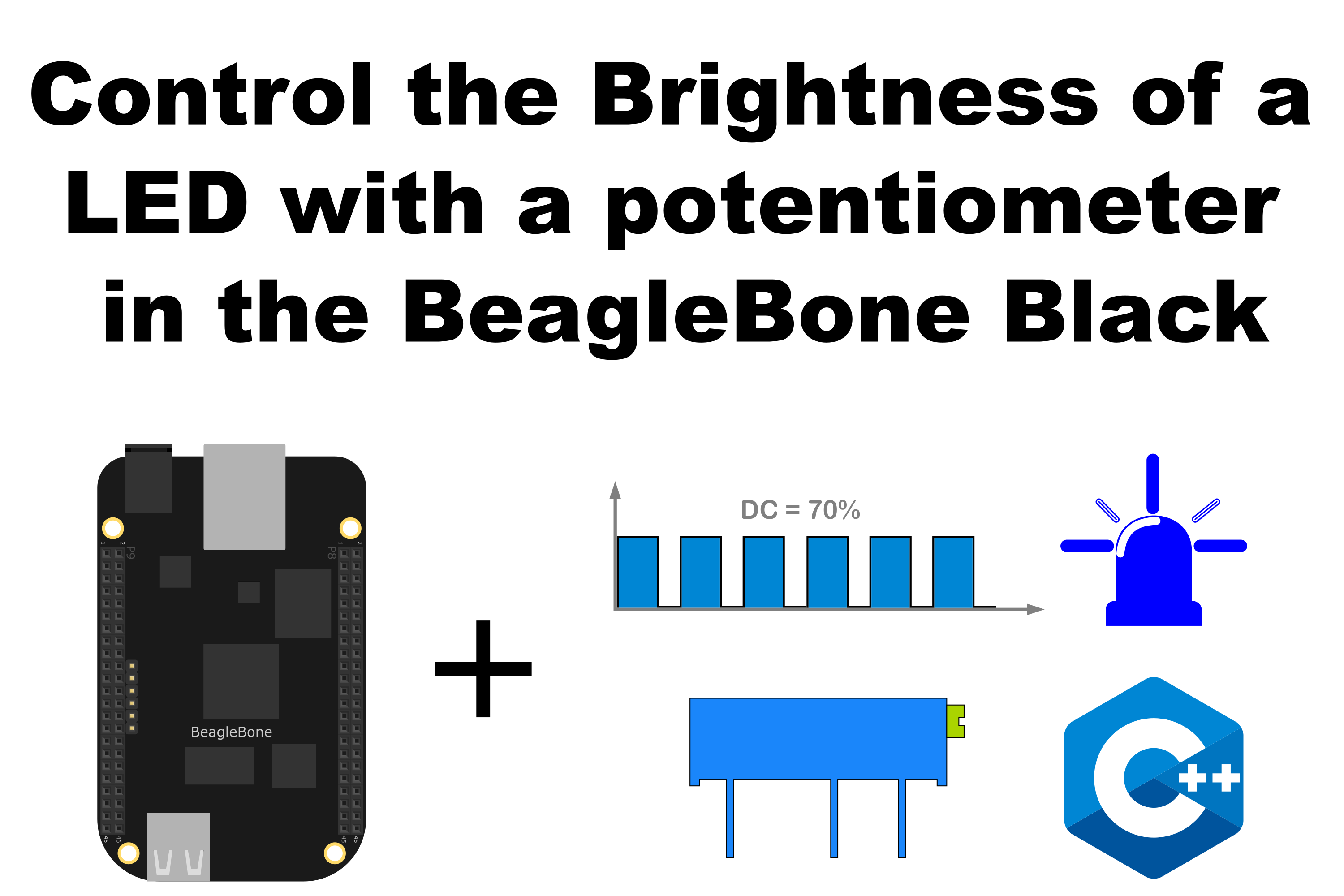
In this post, I show how to control the brightness of a LED with a potentiometer. The LED is wired to a PWM pin and the Potentiometer to an ADC pin. Remembering that the BeagleBone has 7 analog inputs and ADC of 12 bits that let the user to represent an analog signal within a range of 4096 values. It is important to remember that the reference for analog voltage is 1.8V. If the user provides a greater voltage, the BeagleBone could be damaged.
Circuit and components
The circuit can be seen in Figure 1. It consists of a Potentiometer with a power supplied using the analog ground pin 0V located at the pin P9_34 and to analog VDD pin at 1.8V located at the pin P9_32. Finally, its output is connected to the P9_39 pin. On the other hand, the LED is connected to PWM pin P8_13 to see how its brightness can vary according to the potentiometer’s voltage value.
The components are:
- 1 Potentiometer of 1MΩ
- 1 Resistor of 1KΩ
- 1 LED of 3mm
- Jumpers male-male to make the connections
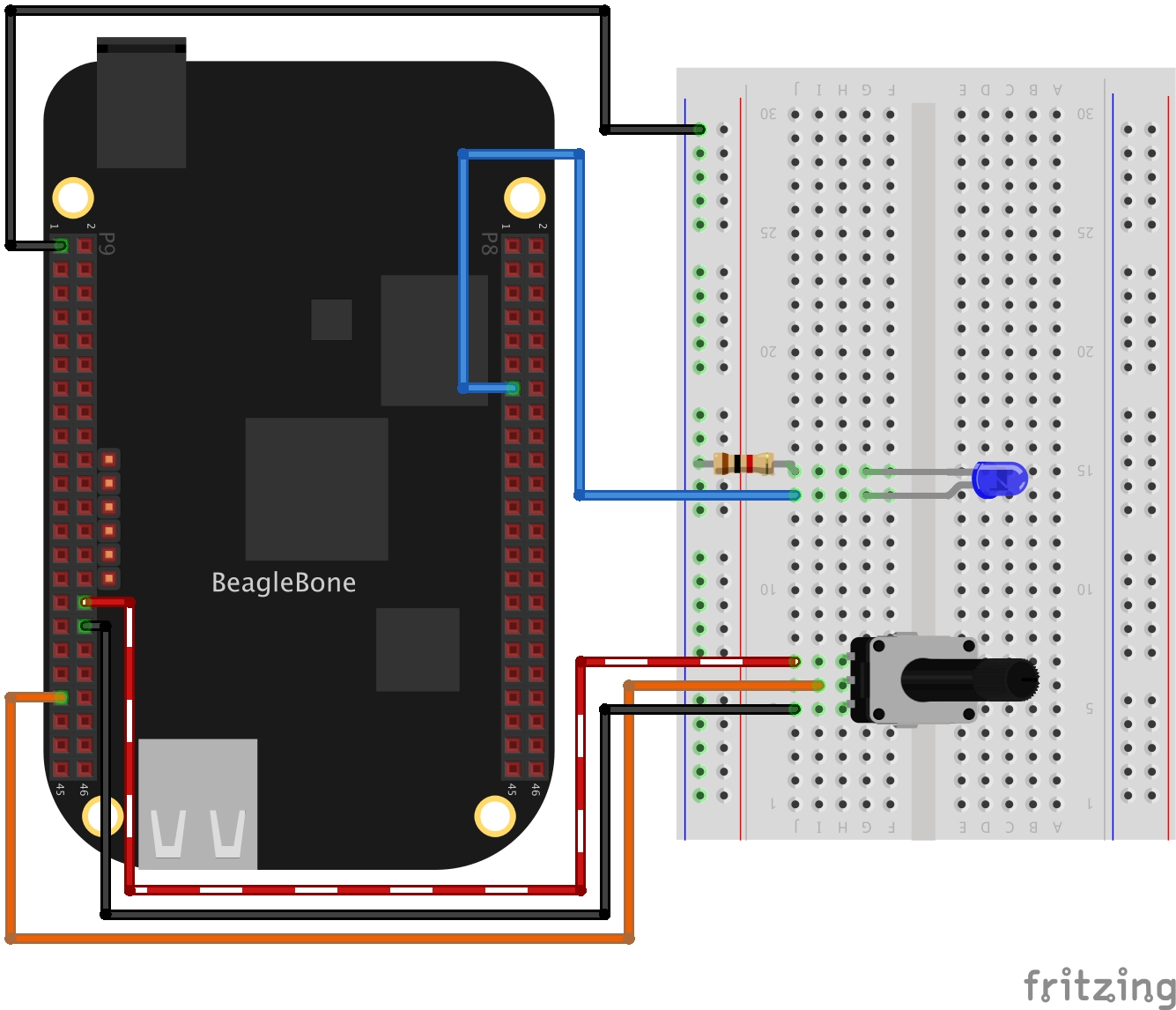
Coding
First, ADC
and PWM
class objects are declared as global variables:
1
2
3
4
// Global ADC pin declaration
ADC adcPin(P9_39);
// Global PWM pin declaration
PWM pwmBlueLedPin(P8_13);
An integer variable is declared and initialized to store the analog value on the pin. You can note the name contains the suffix Out
because this variable is passed by reference to the corresponding method, after in the main program. On the other hand, boolean and integer variables are declared and initialized to stop and keep the execution of the PWM cycle on the pin and the value on this, respectively.
1
2
3
4
// Global variables
bool stopBrightLed = false;
int adcValueOut = 0;
int pwmValue = 0;
A user function is defined to read the analog value (0-4095) on the potentiometer and to write the mapped value (0-100) on the PWM pin. This function is controlled by the boolean variable stopBrightLed
which gives the signal to stop the execution.
1
2
3
4
5
6
7
8
9
10
11
12
13
int BrightLed()
{
while (stopBrightLed == false)
{
adcPin.ReadADC(adcValueOut);
cout << "ADC value on pin: " << adcValueOut << " / ";
pwmValue = adcValueOut / 4095.0 * 100;
cout << "PWM value: " << pwmValue << endl;
pwmBlueLedPin.SetDutyCycle(pwmValue);
Delayms(100);
}
return 0;
}
In the main program a while loop
can be used to wait for a user keypress to stop the continuous sampling method before finishing the program.
1
2
3
4
5
6
7
8
char userInput = '\0';
while (userInput != 'y')
{
message = "Do you want to stop the readings on the pin? Enter 'y' for yes:";
cout << RainbowText(message, "Blue") << endl;
cin >> userInput;
if (userInput == 'y') stopBrightLed = true;
}
Before finishing the program, the PWM value on the pin is cleaned.
1
2
// Clean the pwm value on pin
pwmBlueLedPin.SetDutyCycle(0);
The complete code for this application is shown in the next listing together with its corresponding execution video.
Listing_5.5
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
/******************************************************************************
Listing_5.5.cpp
@wgaonar
03/04/2021
https://github.com/wgaonar/BeagleCPP
Control de brightness of a LED with an analog value from a POT wired to AIN0 (P9_39) in background each 100 milliseconds while polling an key stroke by the user
Class: ADC
******************************************************************************/
#include <iostream>
#include "../../Sources/ADC.h"
#include "../../Sources/PWM.h"
using namespace std;
// Global ADC pin declaration
ADC adcPin(P9_39);
// Global PWM pin declaration
PWM pwmBlueLedPin(P8_13);
// Global variables
bool stopBrightLed = false;
int adcValueOut = 0;
int pwmValue = 0;
int BrightLed()
{
while (stopBrightLed == false)
{
adcPin.ReadADC(adcValueOut);
cout << "ADC value on pin: " << adcValueOut << " / ";
pwmValue = adcValueOut / 4095.0 * 100;
cout << "PWM value: " << pwmValue << endl;
pwmBlueLedPin.SetDutyCycle(pwmValue);
Delayms(100);
}
return 0;
}
int main()
{
string message = "Main program starting here...";
cout << RainbowText(message,"Blue", "White", "Bold") << endl;
// Call the function to read the pin
adcPin.DoUserFunction(&BrightLed);
char userInput = '\0';
while (userInput != 'y')
{
message = "Do you want to stop the readings on the pin? Enter 'y' for yes:";
cout << RainbowText(message, "Blue") << endl;
cin >> userInput;
if (userInput == 'y') stopBrightLed = true;
}
// Clean the pwm value on pin
pwmBlueLedPin.SetDutyCycle(0);
message = "Main program finishes here...";
cout << RainbowText(message,"Blue", "White","Bold") << endl;
return 0;
}
Execution of the program:
Besides, the internal PWM
signal can be seen in the next oscilloscope recording:
Se you in the next post.