Updates to the C++ library for the BeagleBone Black
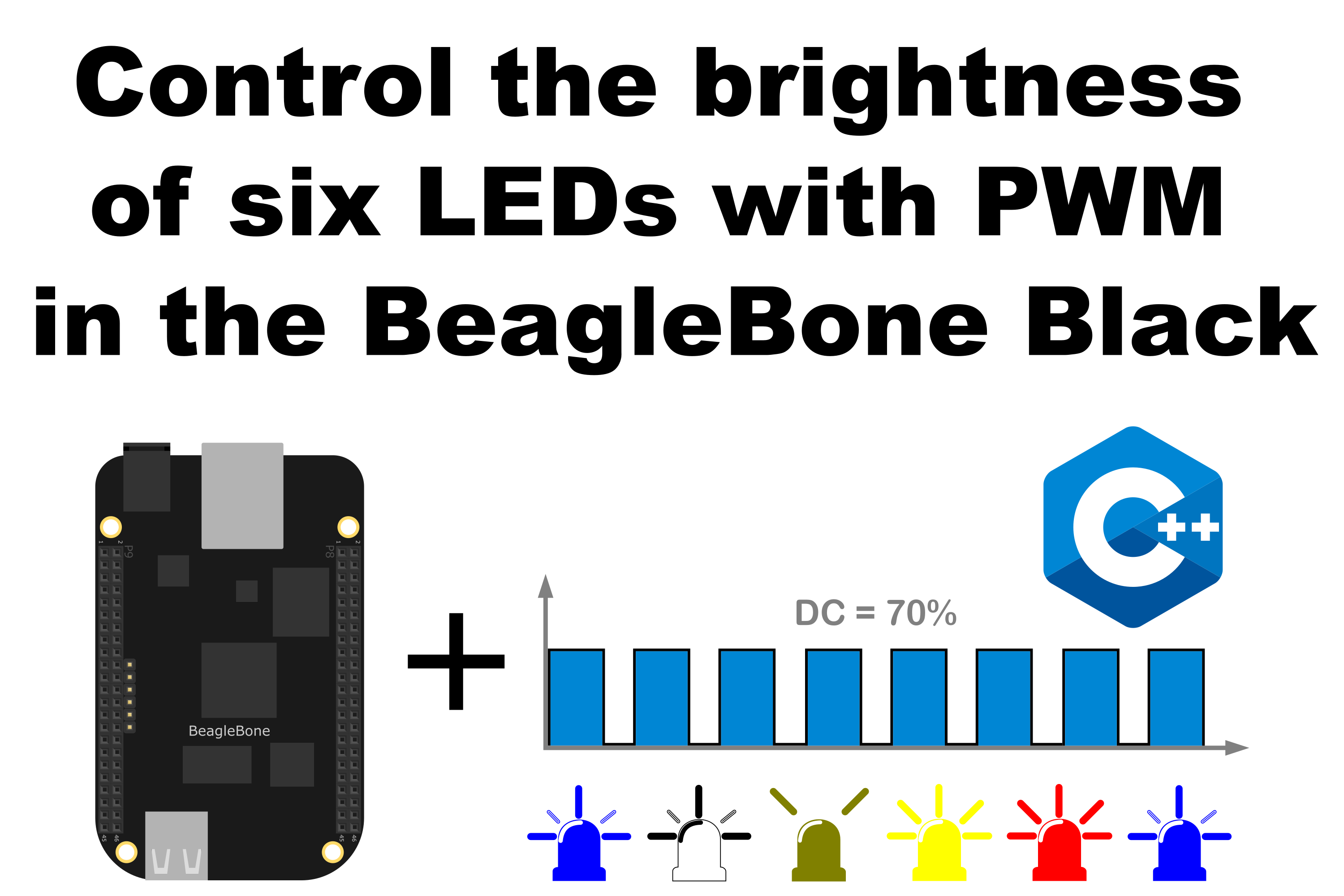
In this post, I use the six PWM pins available on the BeagleBone and show how to work with them in order to control the brightness of six LEDs. Remembering the PWM is a technique that lets us emulate an analog signal in a digital pin. This is done by changing the time that a signal is On in a fixed period of time. The ratio between the time ON and OFF is named duty cycle. The more duty cycle, the more is the “analog” average voltage measured on the digital pin. In this post you can find updated code of the class.
Circuit and components
The circuit can be seen in Figure 1. Please keep in mind that the BeagleBone works at 3.3V and not 5V like microcontrollers as Arduino. It is so muy important to avoid damage to the board, especially when you are working with buttons or digital inputs in general.
The components are:
- 5 Resistors of 1KΩ
- 1 LED
- Jumpers male-male to make the connections
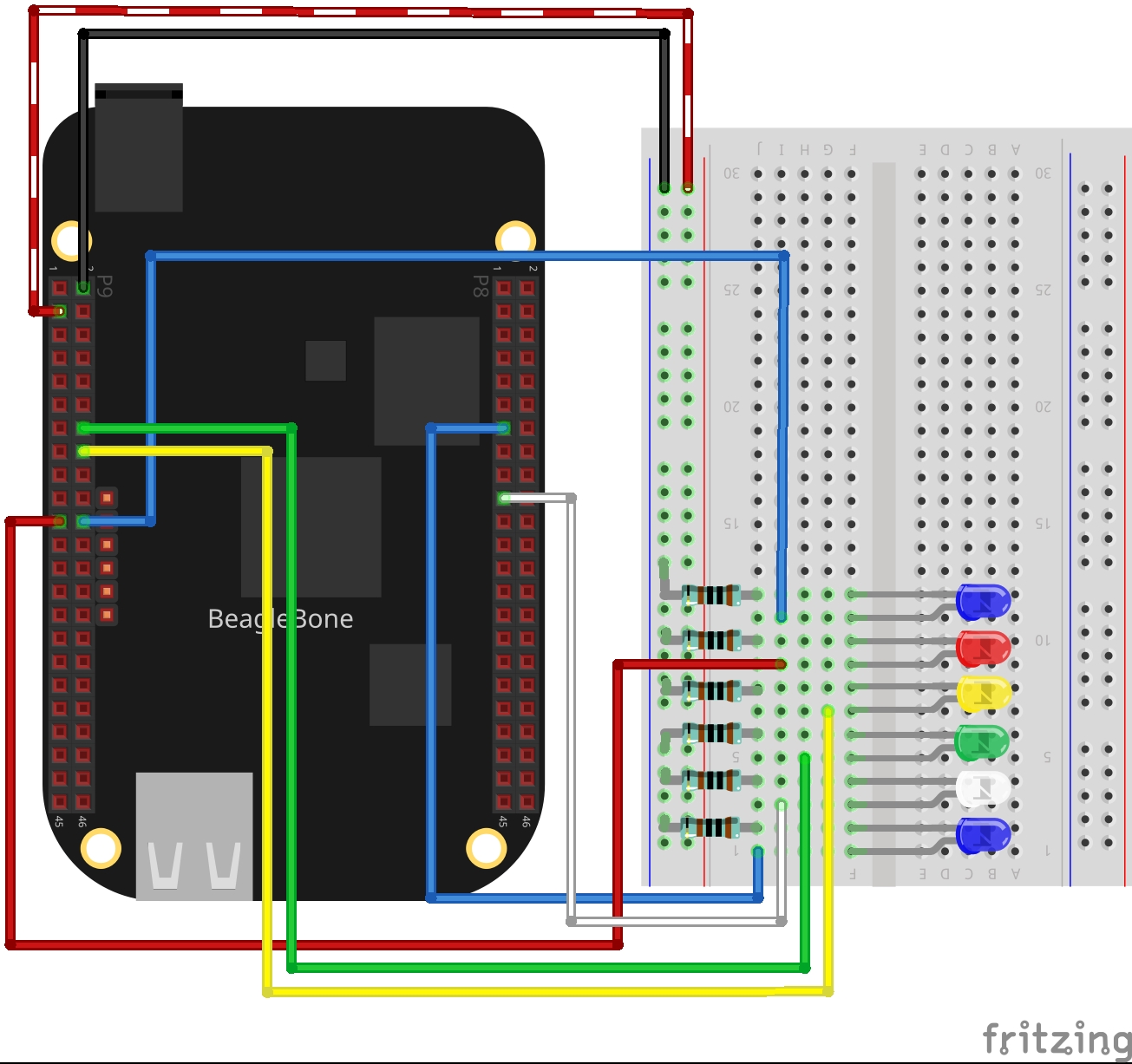
Coding
First of all, each one of the six PWM
class objects is declared:
1
2
3
4
5
6
7
// Global pwm pin declaration
PWM pwmBlueLedPin(P8_13);
PWM pwmWhiteLedPin(P8_19);
PWM pwmGreenLedPin(P9_14);
PWM pwmYellowLedPin(P9_16);
PWM pwmRedLedPin(P9_21);
PWM pwmBlueLedPin2(P9_22);
Secondly, a function to do a “stairs pattern” is defined. This function takes an argument a PWM
object and makes a cycle increasing the duty cycle from 0 to 100 and after, decreasing it from 100 to 0.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
int DoStairs(PWM pwmPin)
{
// Make a 'stairs pattern to up' on the Led
cout << "Doing a stairs pattern to up on a Blue Led..." << endl;
for (int pwmValue = 0; pwmValue <= 100; pwmValue += 10)
{
cout << "Setting a duty cycle of: " << pwmValue << endl;
pwmPin.SetDutyCycle(pwmValue);
pwmPin.Delayms(50);
}
cout << endl;
// Make a 'stairs pattern to down' on the Led
cout << "Doing a stairs pattern to down on a Blue Led..." << endl;
for (int pwmValue = 100; pwmValue >= 0; pwmValue -= 10)
{
cout << "Setting a duty cycle of: " << pwmValue << endl;
pwmPin.SetDutyCycle(pwmValue);
pwmPin.Delayms(50);
}
cout << endl;
return 0;
}
Finally, in the main code the function is called for every PWM pin:
1
2
3
4
5
6
pDoStairs(pwmBlueLedPin);
DoStairs(pwmWhiteLedPin);
DoStairs(pwmGreenLedPin);
DoStairs(pwmYellowLedPin);
DoStairs(pwmRedLedPin);
DoStairs(pwmBlueLedPin2);
The complete code for this application is shown in the next listing together with its corresponding execution video.
Listing_4.2
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
#include <iostream>
#include <cmath>
#include "../../Sources/PWM.h"
using namespace std;
// Global pwm pin declaration
PWM pwmBlueLedPin(P8_13);
PWM pwmWhiteLedPin(P8_19);
PWM pwmGreenLedPin(P9_14);
PWM pwmYellowLedPin(P9_16);
PWM pwmRedLedPin(P9_21);
PWM pwmBlueLedPin2(P9_22);
int DoStairs(PWM pwmPin)
{
// Make a 'stairs pattern to up' on the Led
cout << "Doing a stairs pattern to up on a Blue Led..." << endl;
for (int pwmValue = 0; pwmValue <= 100; pwmValue += 10)
{
cout << "Setting a duty cycle of: " << pwmValue << endl;
pwmPin.SetDutyCycle(pwmValue);
pwmPin.Delayms(50);
}
cout << endl;
// Make a 'stairs pattern to down' on the Led
cout << "Doing a stairs pattern to down on a Blue Led..." << endl;
for (int pwmValue = 100; pwmValue >= 0; pwmValue -= 10)
{
cout << "Setting a duty cycle of: " << pwmValue << endl;
pwmPin.SetDutyCycle(pwmValue);
pwmPin.Delayms(50);
}
cout << endl;
return 0;
}
int main()
{
string message = "Main program starting here...";
cout << RainbowText(message,"Blue", "White", "Bold") << endl;
DoStairs(pwmBlueLedPin);
DoStairs(pwmWhiteLedPin);
DoStairs(pwmGreenLedPin);
DoStairs(pwmYellowLedPin);
DoStairs(pwmRedLedPin);
DoStairs(pwmBlueLedPin2);
message = "Main program finishes here...";
cout << RainbowText(message,"Blue", "White","Bold") << endl;
return 0;
}
Execution of the program:</h2>
Se you in the next post.