Reading an analog value with continuous sampling in the BeagleBone Black
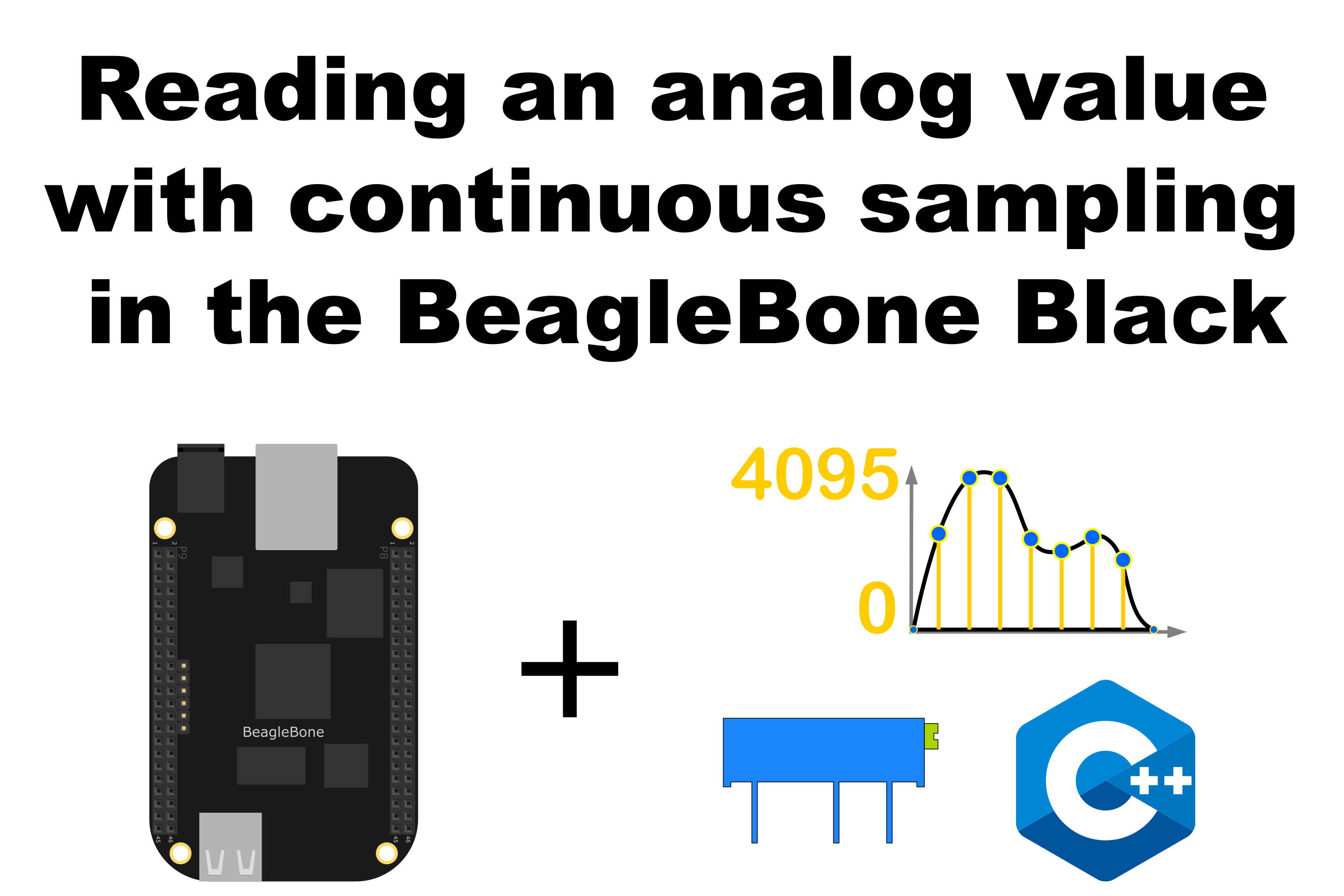
In this post, I show a simple application to read an analog value running in the background with its own thread with the ADC C++ class implementation shown previously in this post. Remembering that the BeagleBone has 7 analog inputs and ADC of 12 bits that let to de user to represent an analog signal within a range of 4096 values. It is important to remember that the reference for analog voltage is 1.8V. If the user provides a greater voltage, the BeagleBone could be damaged.
Circuit and components
The circuit can be seen in Figure 1. It consists of a Potentiometer with a power supplied using the analog ground pin 0V located at the pin P9_34 and to analog VDD pin at 1.8V located at the pin P9_32. Finally, its output is connected to the P9_39 pin.
The components are:
- 1 Potentiometer of 200KΩ
- Jumpers male-male to make the connections
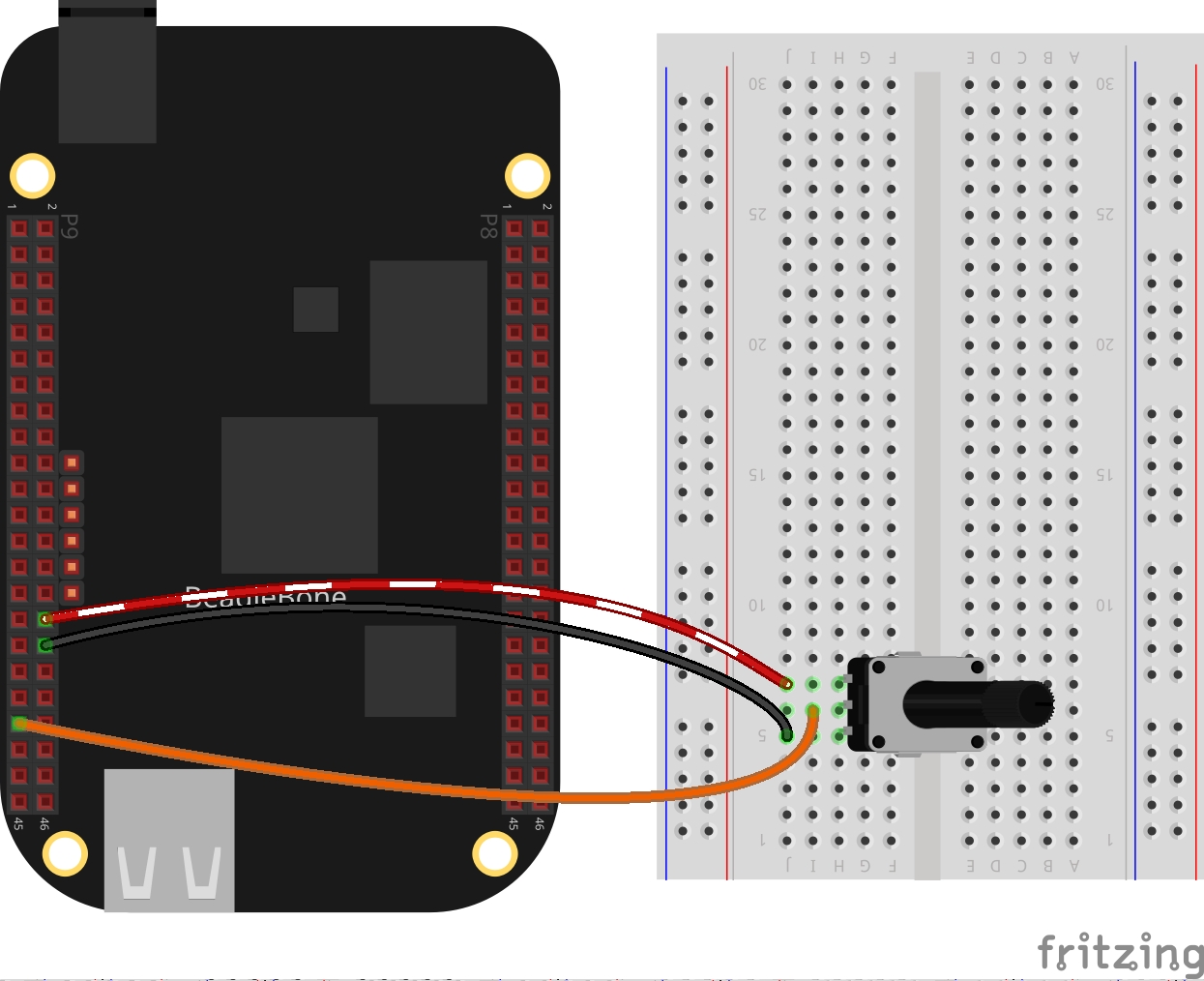
Coding
First an ADC
class object is declared, for example:
1
ADC adcPin(P9_39);
An integer variable is declared and initialized to store the digital value. You can note the name contains the suffix Out
because this variable is passed by reference to the corresponding method, after in the main program.
1
int adcValueOut = 0;
An integer variable is declared and initialized to set the interval time between each reading.
1
int sampleTime = 100;
The continuous sampling of digital values can be obtained through the next class method. It takes two arguments, the first is the variable, passed by reference, to store the digital value and the second is the interval between each reading. The sampling is made in the background through an internal thread execution. Besides, this method does not return any value.
1
adcPin.ReadADCContinuousSampling(adcValueOut,sampleTime);
This method can be stopped through the next line of code:
1
adcPin.StopReadADCContinuousSampling();
In the main program a while loop
can be used to wait for a user key press to stop the continuous sampling method before finishing the program.
1
2
3
4
5
6
7
8
9
10
while (userInput != 'y')
{
message = "Do you want to stop the readings on the pin? Enter 'y' for yes:";
cout << RainbowText(message, "Blue") << endl;
cin >> userInput;
if (userInput == 'y')
{
adcPin.StopReadADCContinuousSampling();
}
}
The complete code for this application is shown in the next listing together with its corresponding execution video.
Listing_5.3
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
#include <iostream>
#include "../../Sources/ADC.h"
using namespace std;
int main()
{
string message = "Main program starting here...";
cout << RainbowText(message,"Blue", "White", "Bold") << endl;
message = "Setting ADC mode on a pin";
cout << RainbowText(message, "Blue") << endl;
ADC adcPin(P9_39);
message = "Read continuously the ADC value on pin";
cout << RainbowText(message, "Blue") << endl;
int adcValueOut = 0;
int sampleTime = 100;
adcPin.ReadADCContinuousSampling(adcValueOut,sampleTime);
char userInput = '\0';
while (userInput != 'y')
{
message = "Do you want to stop the readings on the pin? Enter 'y' for yes:";
cout << RainbowText(message, "Blue") << endl;
cin >> userInput;
if (userInput == 'y')
{
adcPin.StopReadADCContinuousSampling();
}
}
message = "Main program finishes here...";
cout << RainbowText(message,"Blue", "White","Bold") << endl;
return 0;
}
Execution of the program:
Se you in the next post.