Doing a pulse light effect on a LED with PWM in the BeagleBone Black
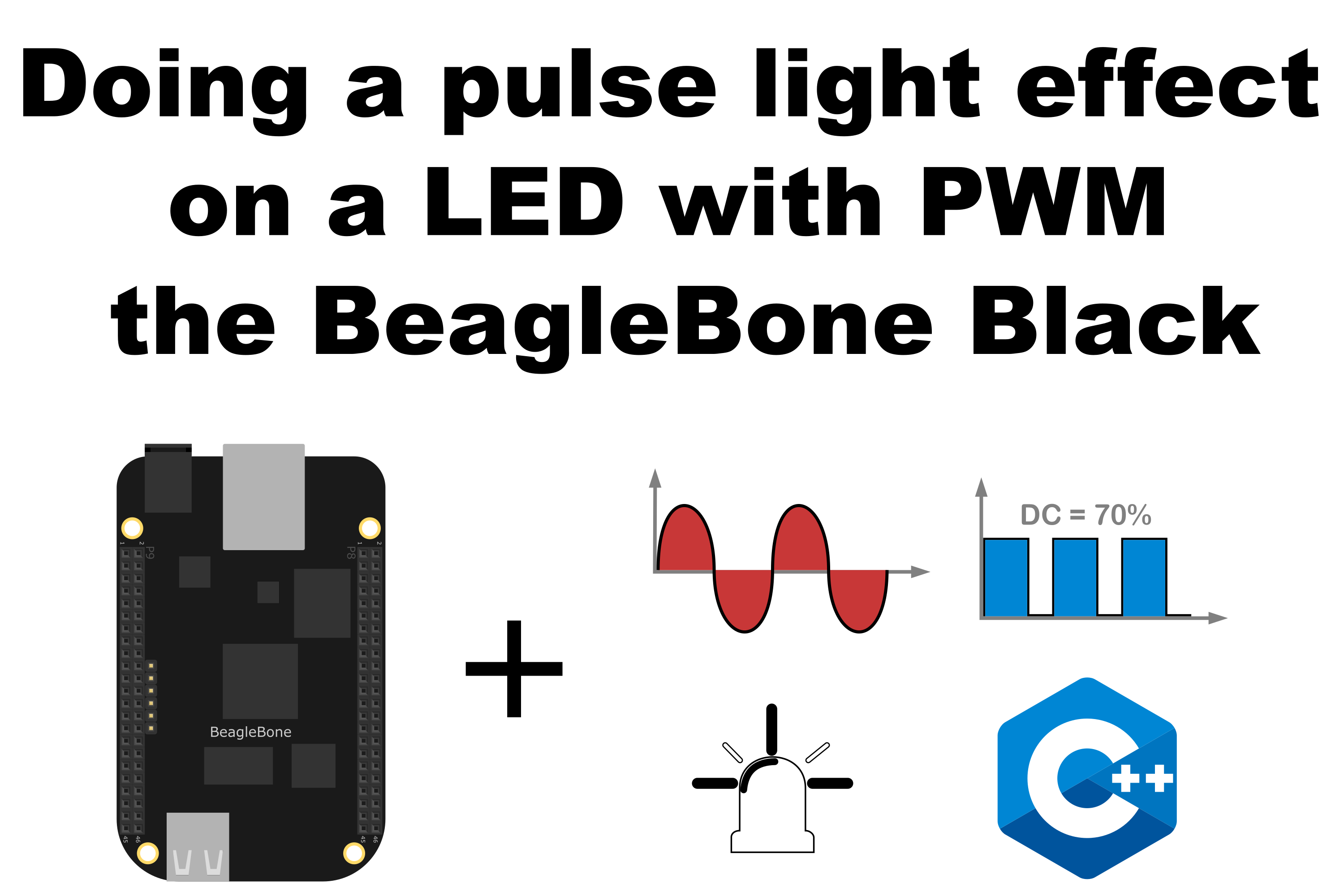
In this post, I start applying the PWM technique to do a light pulse effect on a LED. This is inspired by the work by Sparkfun titled Pulse a LED, where the brightness of a LED is changed using a sine wave, instead of a classic ramp or linear function.
Circuit and components
The circuit can be seen in Figure 1. Please keep in mind that the BeagleBone works at 3.3V and not 5V like microcontrollers as Arduino. It is so important to avoid damage to the board, especially when you are working with buttons or digital inputs in general.
The components are:
- 5 Resistor of 1KΩ
- 1 LED
- Jumpers male-male to make the connections
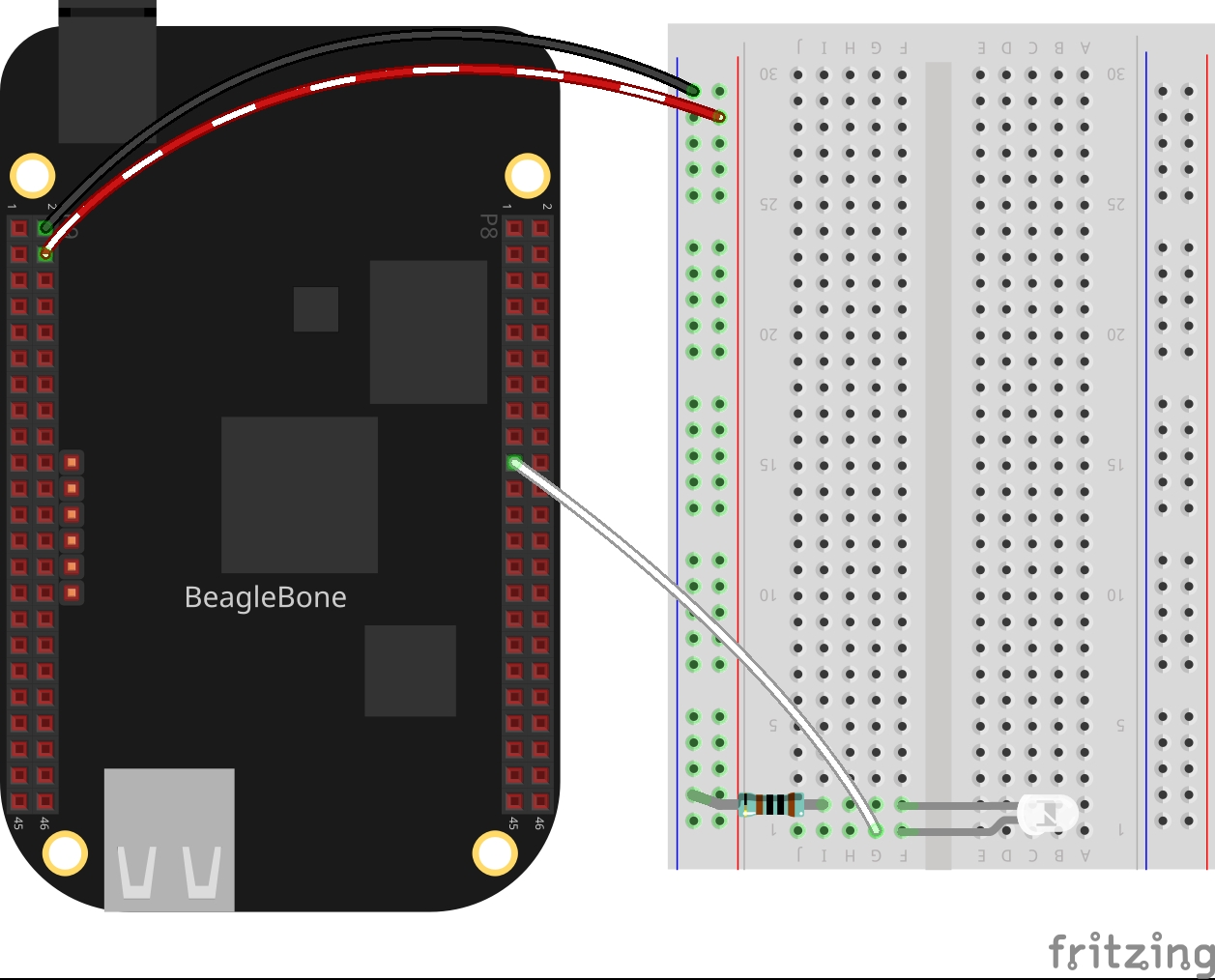
Coding
First an PWM
class object is declared, initializing the pin and its period:
1
2
// Global pwm pin declaration
PWM pwmWhiteLedPin(P8_19,600000);
After, a user function is defined. This function changes the duty cycle using a sine wave on 100 samples in a range of values between 0 and 100. This function uses a flag to stop it when the user presses a specific key.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
bool stopPulse = false;
int PulseLed()
{
while (stopPulse == false)
{
for (float in = 0; in < 6.28; in += 0.0628)
{
float out = sin(in) * 50 + 50;
pwmWhiteLedPin.SetDutyCycle(out);
pwmWhiteLedPin.Delayms(10);
}
}
return 0;
}
In the main code, this PulseLed()
function
is used and a function pointer argument to the PWM class method
DoUserFunction(&)
which inside the class
constructs a thread to run this function in parallel with the main program.
1
2
// Call the function to pulse the LED
pwmWhiteLedPin.DoUserFunction(&PulseLed);
Finally, the main program is always waiting for the press of “y” key to finish the program stopping the PulseLed()
function and setting the true the flag to finish the execution of the DoUserFunction(&)
method.
1
2
3
4
5
6
7
8
9
10
11
12
char userInput = '\0';
while (userInput != 'y')
{
message = "Do you want to stop the pulse on the led? Enter 'y' for yes: ";
cout << RainbowText(message, "Violet");
cin >> userInput;
if (userInput == 'y')
{
stopPulse = true;
pwmWhiteLedPin.StopUserFunction();
}
}
The complete code for this application is shown in the next listing together with its corresponding execution video.
Listing_4.2
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
#include <iostream>
#include <cmath>
#include "../../Sources/PWM.h"
using namespace std;
// Global pwm pin declaration
PWM pwmWhiteLedPin(P8_19,600000);
bool stopPulse = false;
int PulseLed()
{
while (stopPulse == false)
{
for (float in = 0; in < 6.28; in += 0.0628)
{
float out = sin(in) * 50 + 50;
pwmWhiteLedPin.SetDutyCycle(out);
pwmWhiteLedPin.Delayms(10);
}
}
return 0;
}
int main()
{
string message = "Main program starting here...";
cout << RainbowText(message,"Blue", "White", "Bold") << endl;
message = "Pulse a white led";
cout << RainbowText(message, "White") << endl;
// Call the function to pulse the LED
pwmWhiteLedPin.DoUserFunction(&PulseLed);
char userInput = '\0';
while (userInput != 'y')
{
message = "Do you want to stop the pulse on the led? Enter 'y' for yes: ";
cout << RainbowText(message, "Violet");
cin >> userInput;
if (userInput == 'y')
{
stopPulse = true;
pwmWhiteLedPin.StopUserFunction();
}
}
message = "Main program finishes here...";
cout << RainbowText(message,"Blue", "White","Bold") << endl;
return 0;
}
Execution of the program:
Se you in the next post.