Control the brightness of a LED with PWM in the BeagleBone Black
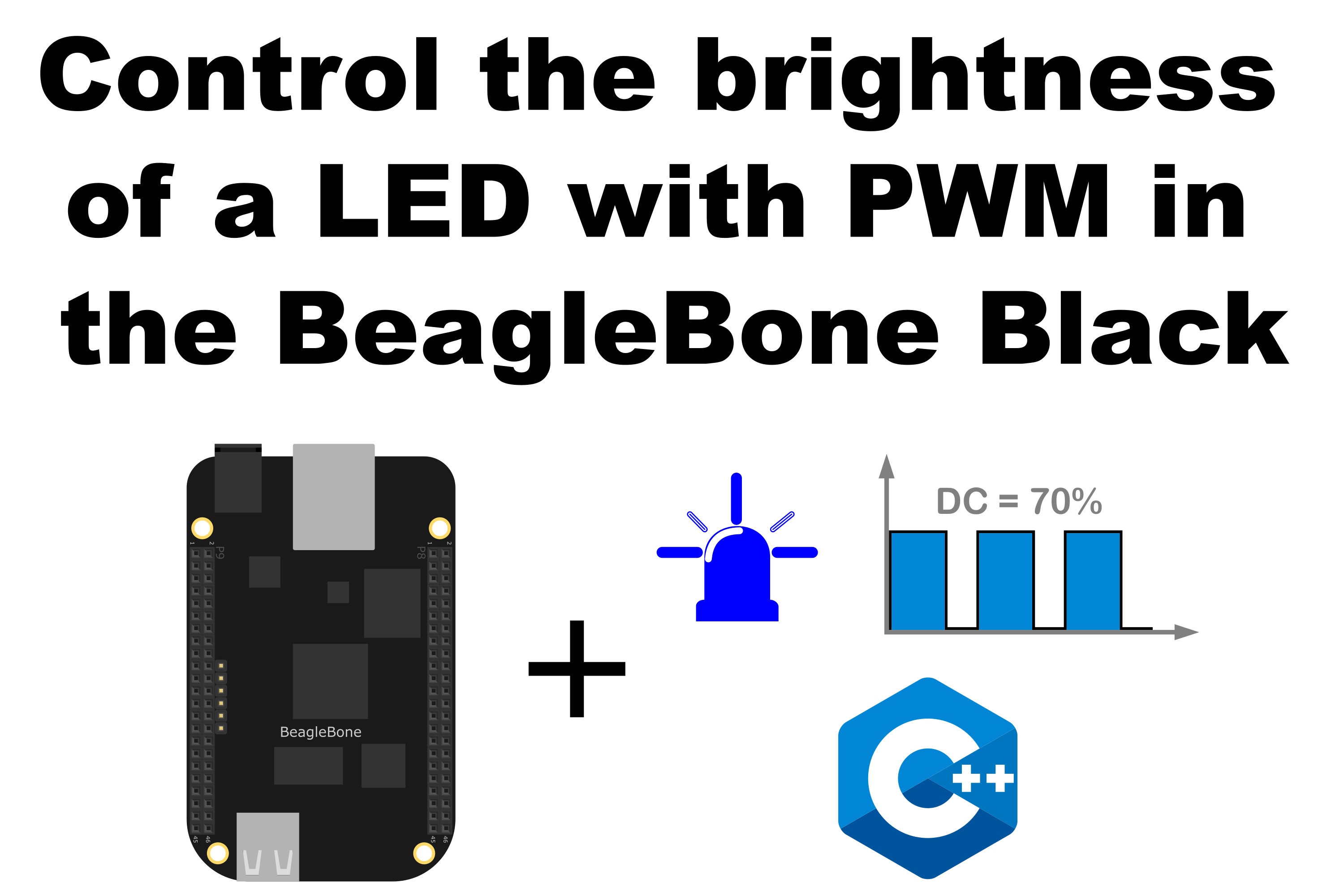
In this post, I start with the PWM technique applied to control the brightness of a LED. Remembering the PWM is a technique that lets us emulate an analog signal in a digital pin. This is done by changing the time that a signal is On in a fixed period of time. The ratio between the time ON and OFF is named duty cycle. The more duty cycle, the more is the “analog” average voltage measured on the digital pin. In
this post You can find the complete code of the class.
Circuit and components
The circuit can be seen in Figure 1. Please keep in mind that the BeagleBone works at 3.3V and not 5V like microcontrollers as Arduino. It is so important to avoid damage to the board, especially when you are working with buttons or digital inputs in general.
The components are:
- 5 Resistor of 1KΩ
- 1 LED
- Jumpers male-male to make the connections
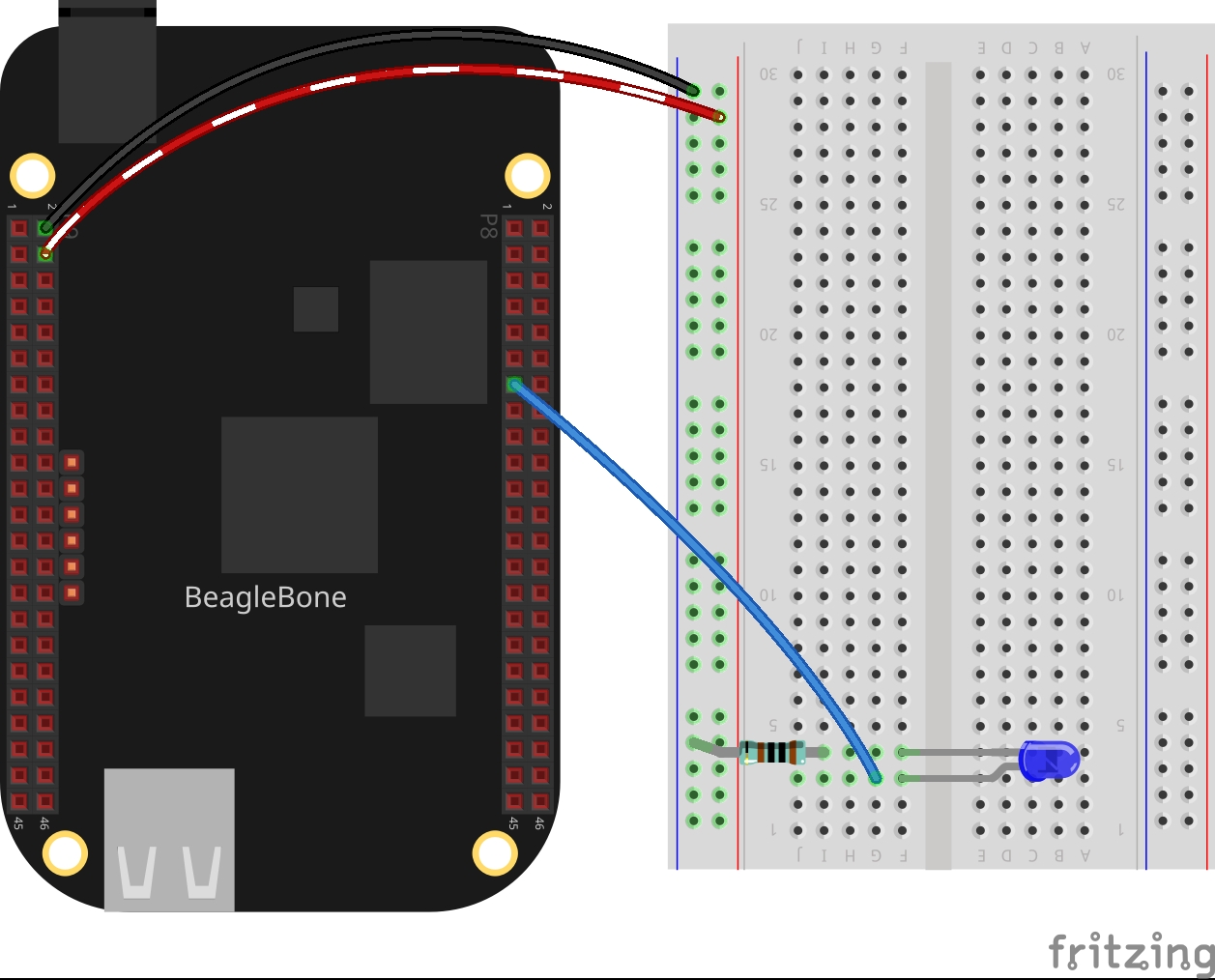
Coding
First an PWM
class object is declared, for example:
1
PWM pwmBlueLedPin(P8_13);
After, a variable is declared, initialized, and used to set the initial pwm value.
1
2
int pwmValue = 50;
pwmBlueLedPin.SetDutyCycle(pwmValue);
Finally, this PWM value can be updated in this manner:
1
pwmBlueLedPin.SetDutyCycle(pwmValue += 10);
The complete code for this application is shown in the next listing together with its corresponding execution video.
Listing_4.1
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
#include <iostream>
#include "../../Sources/PWM.h"
using namespace std;
int main()
{
string message = "Main program starting here...";
cout << RainbowText(message,"Blue", "White", "Bold") << endl;
message = "Setting a PWM mode on a blue led";
cout << RainbowText(message, "Blue") << endl;
PWM pwmBlueLedPin(P8_13);
int pwmValue = 50;
message = "Set a pwm duty cycle of 50%' on a blue led and wait 1 second";
cout << RainbowText(message, "Violet") << endl;
pwmBlueLedPin.SetDutyCycle(pwmValue);
message = "If you want to stop the program, enter 'y' for yes";
cout << RainbowText(message, "Blue") << endl;
message = "Or enter 'w' for increase brightness or 's' for decrease it";
cout << RainbowText(message, "Blue") << endl;
char userInput = '\0';
while (userInput != 'y')
{
message = "Enter an option 'y', 'w', 's': ";
cout << RainbowText(message, "Blue");
cin >> userInput;
switch (userInput)
{
case 'w':
pwmBlueLedPin.SetDutyCycle(pwmValue += 10);
break;
case 's':
pwmBlueLedPin.SetDutyCycle(pwmValue -= 10);
break;
default:
break;
}
}
message = "Main program finishes here...";
cout << RainbowText(message,"Blue", "White","Bold") << endl;
return 0;
}
Execution of the program:
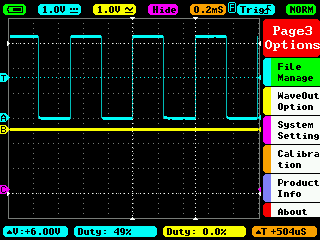
Se you in the next post.