Reading a button with the BeagleBone Black
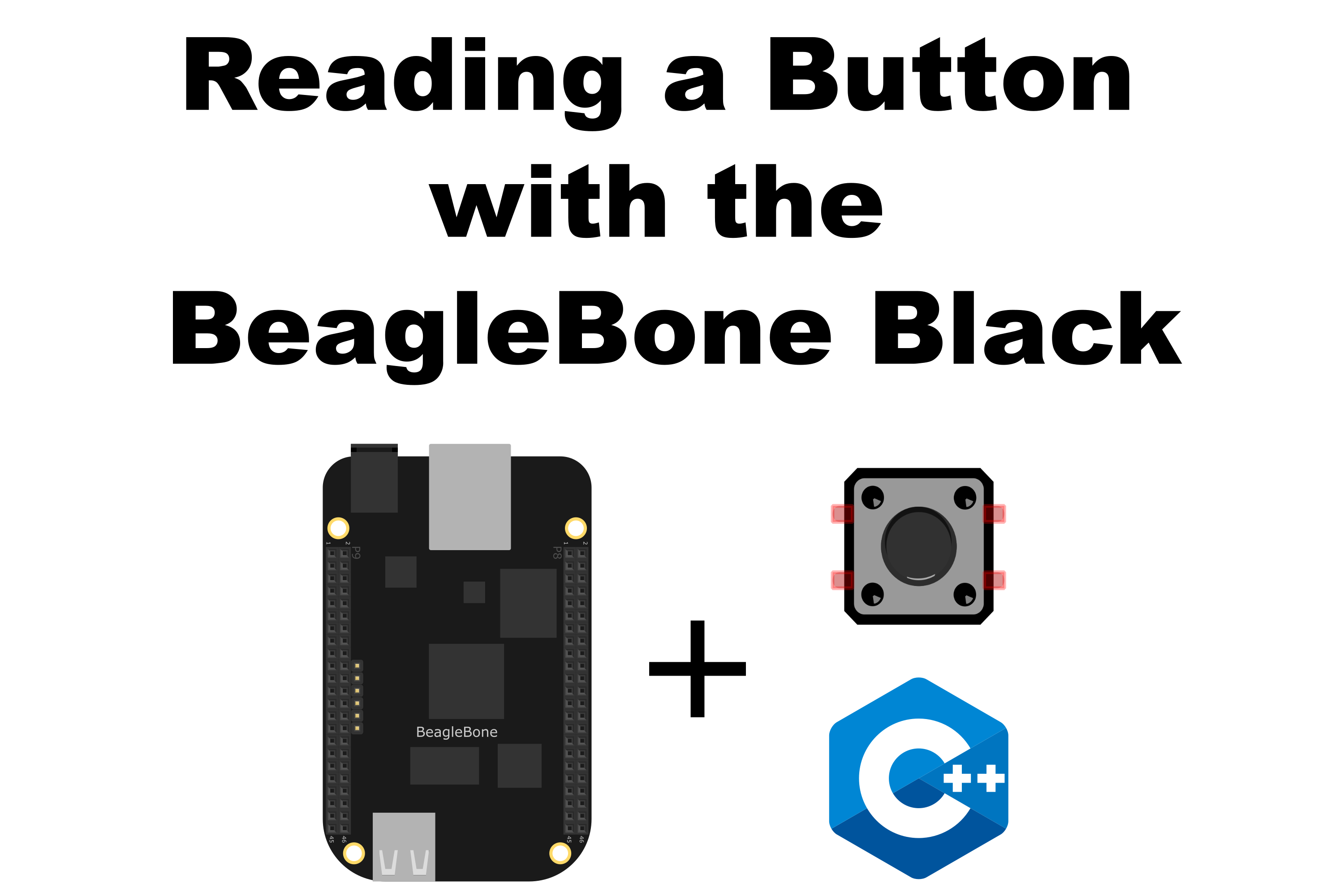
In this post, I will show you how to read the input from a button and turn a led On each time it will be pressed. I will be using the C++ library described in the post BeagleBone and C++ to access and control the general purpose digital pins (GPIO) pins of the BeagleBone.
Circuit and components
The circuit can be seen in Figure 1. Please keep in mind that the BeagleBone works at 3.3V> and not 5V like microcontrollers as Arduino. It is so muy important to avoid damage to the board, specially when your are working with buttons or digital inputs in general.
The components are:
- 1 Red Led of 3mm
- 1 Resistor of 1KΩ
- 1 Resistor of 10KΩ
- 1 Push button of 12mm
- Jumpers male-male to make the connections
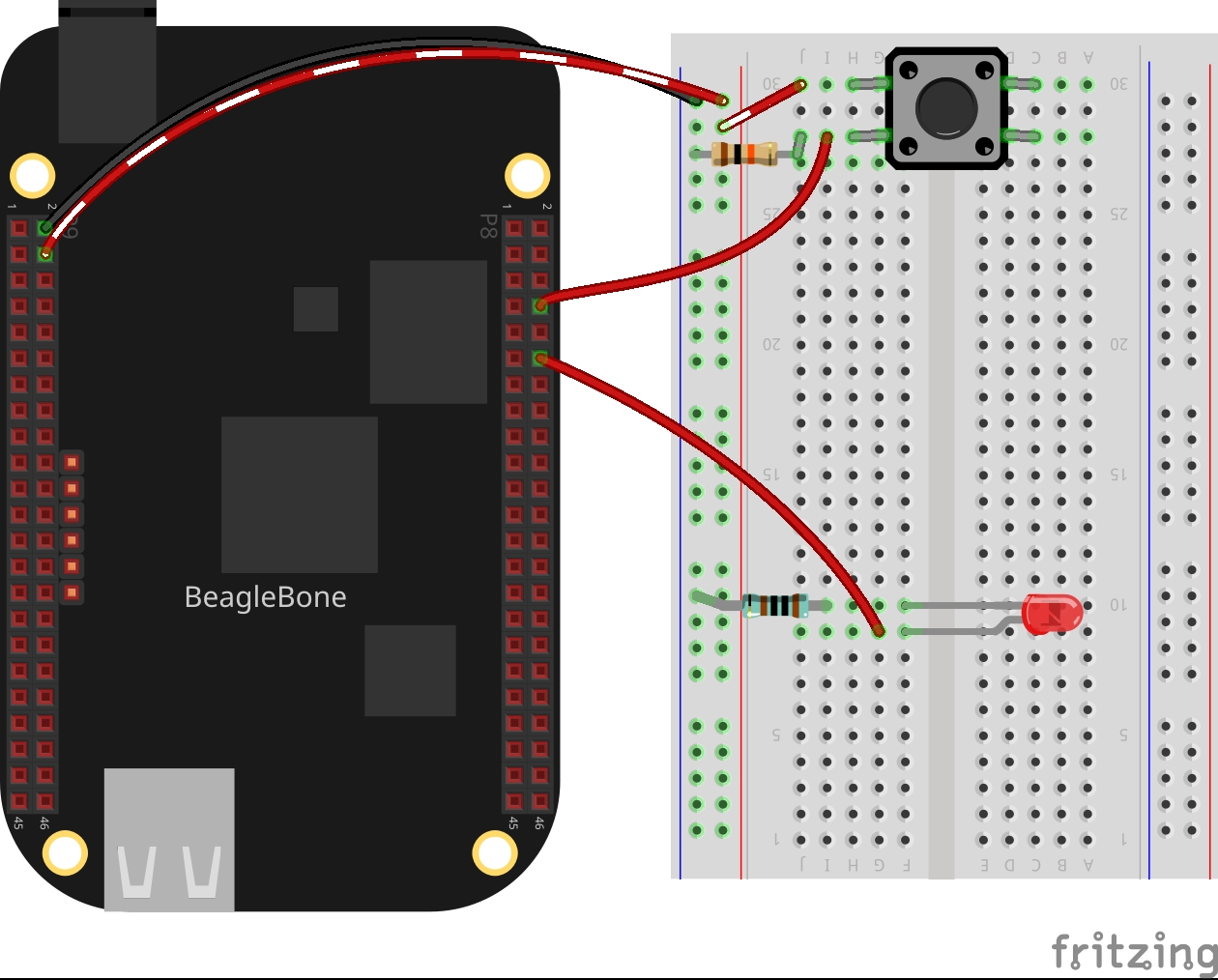
Coding
I coded some lines and have used functions available in the header file the GPIO.h The functions are:
digitalWrite()
digitalRead()
delayms()
The first part of the code defines the pins to be used as input from a button and output for the Led, P8_08 and P8_12, respectively:
1
2
GPIO buttonPin(P8_08, INPUT);
GPIO ledPin(P8_12, OUTPUT);
In the second part of the code, a counter is used to record each time the button is pressed to a maximum of 10 times. In this case, the while loop finishes and then the main program.
Inside this while loop, there are another two while loops. The first waits until the button is pressed, immediately the Led is turned On and the counter is incremented. After that, the second loop waits until the button is released to turn the Led Off.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
int count = 0;
while (count < 10)
{
while (buttonPin.digitalRead() == LOW);
count++;
cout << "The button was pressed, turning the led ON "
<< rainbowText(to_string(count), "Red") << " times" << endl;
ledPin.digitalWrite(HIGH);
while (buttonPin.digitalRead() == HIGH)
ledPin.delayms(10);
cout << "The button was released, turning the led OFF" << endl << endl;
ledPin.digitalWrite(LOW);
ledPin.delayms(10);
}
Listing_1.2
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
#include <iostream>
#include "GPIO.h"
using namespace std;
int main()
{
string message = "Main program starting here...";
cout << rainbowText(message,"Blue", "White", "Bold") << endl;
GPIO buttonPin(P8_08, INPUT);
GPIO ledPin(P8_12, OUTPUT);
int count = 0;
while (count < 10)
{
while (buttonPin.digitalRead() == LOW);
count++;
cout << "The button was pressed, turning the led ON "
<< rainbowText(to_string(count), "Red") << " times" << endl;
ledPin.digitalWrite(HIGH);
while (buttonPin.digitalRead() == HIGH)
ledPin.delayms(10);
cout << "The button was released, turning the led OFF" << endl << endl;
ledPin.digitalWrite(LOW);
ledPin.delayms(10);
}
message = "Main program finishes here...";
cout << rainbowText(message,"Blue", "Bold") << endl;
return 0;
}
Execution of the program
Se you in the next post.