Turning a Led On / Off with the BeagleBone Black
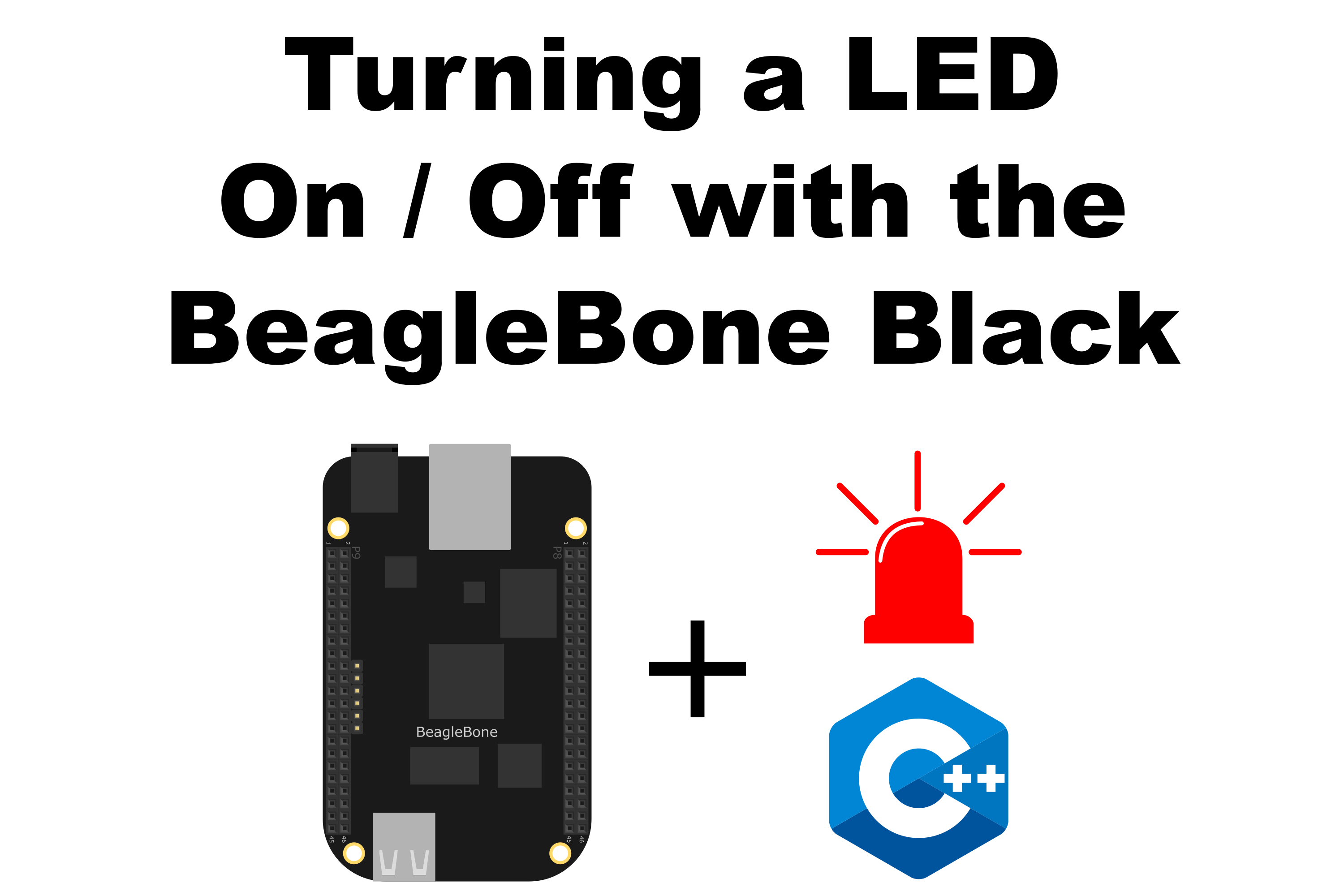
In this post I will show you how to turn a led On and Off using the C++ library described in the post Beaglebone and C++ to access and control the general purpose digital pins (GPIO) pins of the BeagleBone.
Circuit and components
The circuit can be seen in Figure 1. Please keep in mind that the BeagleBone works at 3.3V and not 5V like microcontrollers as Arduino. It is so muy important to avoid damage to the board. The components are:
- 1 Red Led of 3mm
- 1 Resistor of 1 KΩ
- Jumpers male-male to make the connections
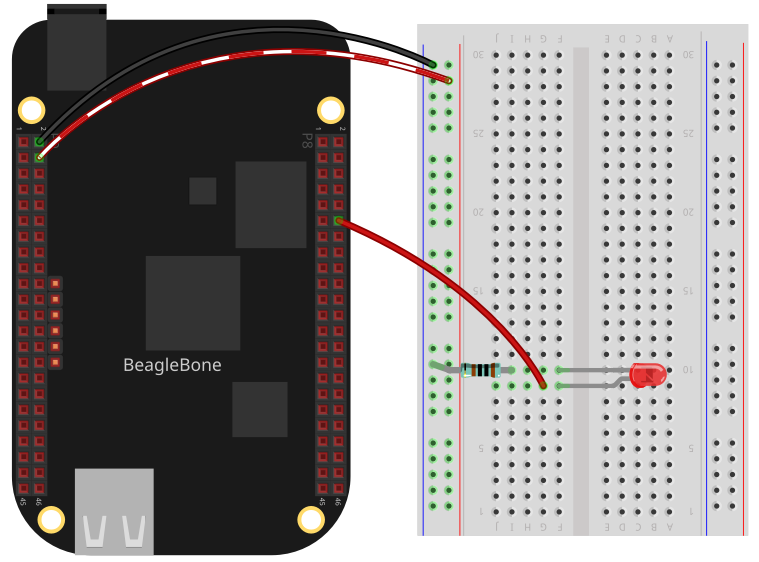
Coding
I coded some lines and have used functions available in GPIO.h header file:
digitalWrite()
digitalRead()
delayms()
The first part of the code defines the pin to be used as an output, in this case, the P8_12:
1
GPIO ledPin(P8_12, OUTPUT);
In the second part of the code, a for loop is used to turn on and off the Led 10 times with an interval of 1000 ms between each on and off cycles, i.e., to make a blinking pattern on the Led:
1
2
3
4
5
6
7
8
for (int i = 0; i < 10; i++)
{
cout << "Blinking " << i+1 << " times out of " << 10 << endl;
ledPin.digitalWrite(HIGH);
ledPin.delayms(1000);
ledPin.digitalWrite(LOW);
ledPin.delayms(1000);
}
Listing_1.1
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
/* Listing_1.1: ledOn.cpp */
#include <iostream>
#include "GPIO.h"
using namespace std;
int main()
{
string message = "Main program starting here...";
cout << rainbowText(message,"Blue", "Bold") << endl;
GPIO ledPin(P8_12, OUTPUT);
for (int i = 0; i < 10; i++)
{
cout << "Blinking " << i+1 << " times out of " << 10 << endl;
ledPin.digitalWrite(HIGH);
ledPin.delayms(1000);
ledPin.digitalWrite(LOW);
ledPin.delayms(1000);
}
message = "Main program finishes here...";
cout << rainbowText(message,"Blue", "Bold") << endl;
return 0;
}
Execution of the program
Se you in the next post.